Determining Whether Your Javascript Object is of Type X
There are times when you’re interested in knowing additional details about the Javascript Object that you’re handling, such as whether you’re handling an Array
, Date
, or standard Object
. However, the typeof
operator, when used against these different objects, returns the following results:
console.log(typeof [1,2,3]) // returns 'object'
console.log(typeof {hello: 'world'}) // returns 'object'
console.log(typeof new Date()) // returns 'object'
Why?
A major pitfall of using the typeof
operator in order to determine the type of objects such as Array
and Date
is that they return 'object’
. For more details, you can view the spec here, but the tl;dr is that all objects except functions and “non-standard exotic”, non-callable objects will return object
when passed to typeof
.
So how do I know what object I’m dealing with?
Fortunately, there’s a way to get values such as 'array'
, 'object'
, 'date'
, etc. from those objects, and that’s where Object.prototype.toString.call()
comes in.
How does it work?
The toString
method is available to all objects that are children of Object
, which Array
and Date
are. The inherited behaviour of toString
, where [object <type>]
is returned, is usually overridden by the more specialized objects. For instance, when the toString
method is called from a Date
object, we would get “Tue Apr 10 2018 09:23:37 GMT-0400 (EDT)”
.
In order to get [object Date]
as opposed to the date as a string, you would need to use the call
method in order to to make the Date
object the this
value for the Object.prototype.toString
function. This is important given how Object.prototype.toString
evaluates what to return when called:
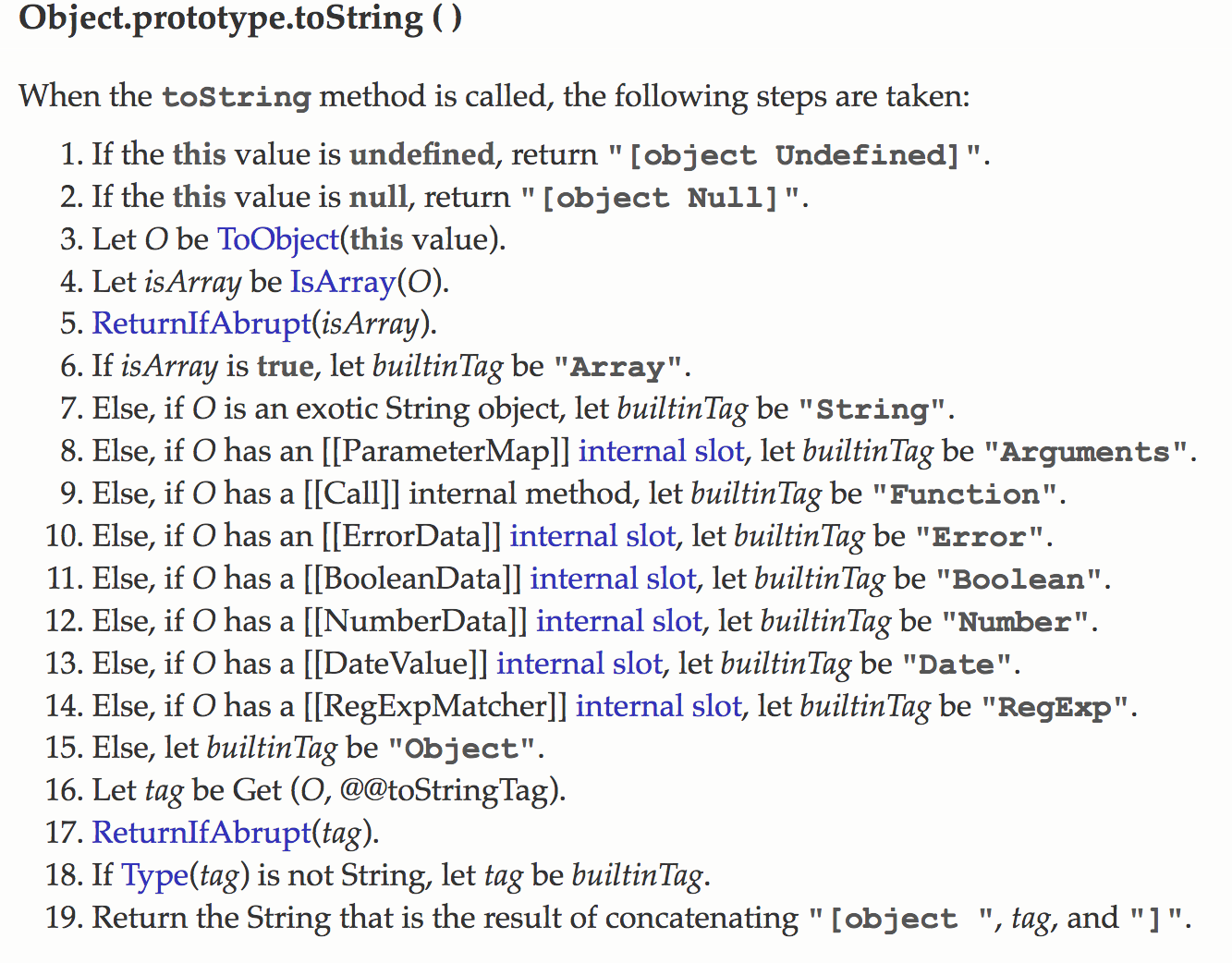
In other words, going back to our example from earlier, by using Object.prototype.toString.call
to determine the type of our objects, we would get the following results:
console.log(Object.prototype.toString.call([1,2,3])); // Returns "[object Array]"
console.log(Object.prototype.toString.call({hello: 'world'})); // Returns "[object Object]"
console.log(Object.prototype.toString.call(new Date())); // Returns "[object Date]"
How can I determine whether my object is a particular class type?
The instanceof
operator can help you here. You can learn more on the MDN page for this operator.
Wrapping up
Object.prototype.toString.call
can be used to create a small utility function in your project that serves as an alternative to typeof
similar to what’s seen in this great blog post. This will remove the need to repeatedly write code such as Object.prototype.toString.call(anArrayObj) === “[object Array]”
.
Happy coding everyone!
Resources used for this article
- https://javascriptweblog.wordpress.com/2011/08/08/fixing-the-javascript-typeof-operator/ (this one was extremely useful, a big source of inspiration for this article, and helped further my understanding of the short comings of typeof — would recommend giving this a read!)
- https://www.ecma-international.org/ecma-262/8.0/index.html#sec-object.prototype.tostring
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/instanceof
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/typeof
- https://www.ecma-international.org/ecma-262/6.0/#sec-typeof-operator
- https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/toString
- https://www.ecma-international.org/ecma-262/6.0/#sec-built-in-exotic-object-internal-methods-and-slots
- https://www.ecma-international.org/ecma-262/6.0/#sec-typeof-operator
- https://www.ecma-international.org/ecma-262/6.0/#sec-function.prototype.call
- https://www.ecma-international.org/ecma-262/6.0/#sec-call
- https://www.ecma-international.org/ecma-262/6.0/#sec-ecmascript-function-objects-call-thisargument-argumentslist
- https://www.ecma-international.org/ecma-262/6.0/#sec-ordinarycallbindthis
- https://www.ecma-international.org/ecma-262/6.0/#sec-toobject
- https://www.ecma-international.org/ecma-262/6.0/#sec-object.prototype.tostring
Member discussion